반응형
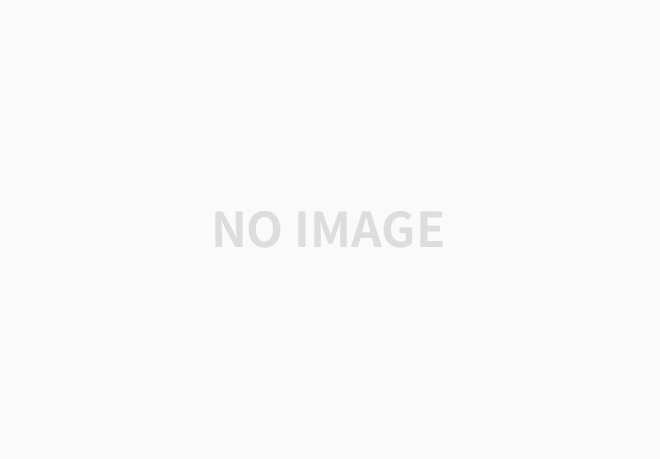
map, filter, reduce
const products = [
{name: '안녕', price: 12000},
{name: '하세요', price: 15000},
{name: '저는', price: 20000},
{name: '지니', price: 25000},
{name: '입니다', price: 15000}
];
map
map 로직
const map = (f, iter) => {//f 라는 함수를 받아서 어떤값을 수집할 것인지 위임한다.(추상화)
let res = [];
for (const a of iter) {
res.push(f(a));
}
return res;
};
- 고차함수이다.
/////////////es5 ///////////////
let names = [];
for (const p of products) {
names.push(p.name); // 직접적으로 코딩을 함
}
log(names);
/////////////es6 ///////////////
///////////위의 내용을 간단하게 작성
log(map(p => p.name, products));
- map 함수의 보조함수를 통해서 products안에 있는 1:1로 매핑되는 값을 전달하겠다는 보조 함수 전달
map의 다형성
map 함수는 이터러블 프로토콜을 따르고 있음
log(map(a => a * a, gen()));
let m = new Map();
m.set('a', 10);
m.set('b', 20);
log(new Map(map(([k, a]) => [k, a * 2], m)));
- 새롭게 map을 받아서 value를 조작할 수 있음
Filter
Filter 로직
const filter = (f, iter) => {
let res = [];
for (const a of iter) {
if (f(a)) res.push(a);
}
return res;
};
/////////////////es5/////////////////
let under20000 = [];
for (const p of products) {
if (p.price < 20000) under20000.push(p);
}
log(...under20000);
/////////////////es6/////////////////
log(...filter(p => p.price < 20000, products));
Reduce
값을 축약하는 함수
array 를 모든 데이터를 축약해서 원하는 새로운 값(함수에 따른 새로운 값) 을 만든다.
Reduce 로직
const reduce = (f, acc, iter) => {
if (!iter) {
iter = acc[Symbol.iterator]();
acc = iter.next().value;
}
for (const a of iter) {
acc = f(acc, a);
}
return acc;
};
const nums = [1, 2, 3, 4, 5];
/////////////////es5/////////////////
let total = 0;
for (const n of nums) {
total = total + n;
}
/////////////////es6/////////////////
const add = (a, b) => a + b;
log(reduce(add, 0, [1, 2, 3, 4, 5]));
// 15
//위의 내용 실행 하는 순서는 아래와 같다. 재귀적으로 실행
log(add(add(add(add(add(0, 1), 2), 3), 4), 5));
// 15
log(reduce(add, [1, 2, 3, 4, 5])); //시작하는 값 생략
// 15
reduce 를 통해 어떻게 축약하나?
모든 가격 다 더하기
reduce((totalprice,product) => total_price + product.price, 0 , products);
짬뽕해서 사용해보기
const add = (a, b) => a + b;
reduce(
add,
map(p => p.price ,
filter(p => p.price <23, products)));
반응형
'프로그래밍 언어 > JavaScript' 카테고리의 다른 글
vanlia javascript 에서 router 구현 (0) | 2021.07.17 |
---|---|
webpack (0) | 2021.07.16 |
ES6 이터러블 /이터레이터, 리스트 순회 (0) | 2021.07.03 |
promise / async await (0) | 2021.06.29 |
javascript 콜백 promise (0) | 2020.04.10 |